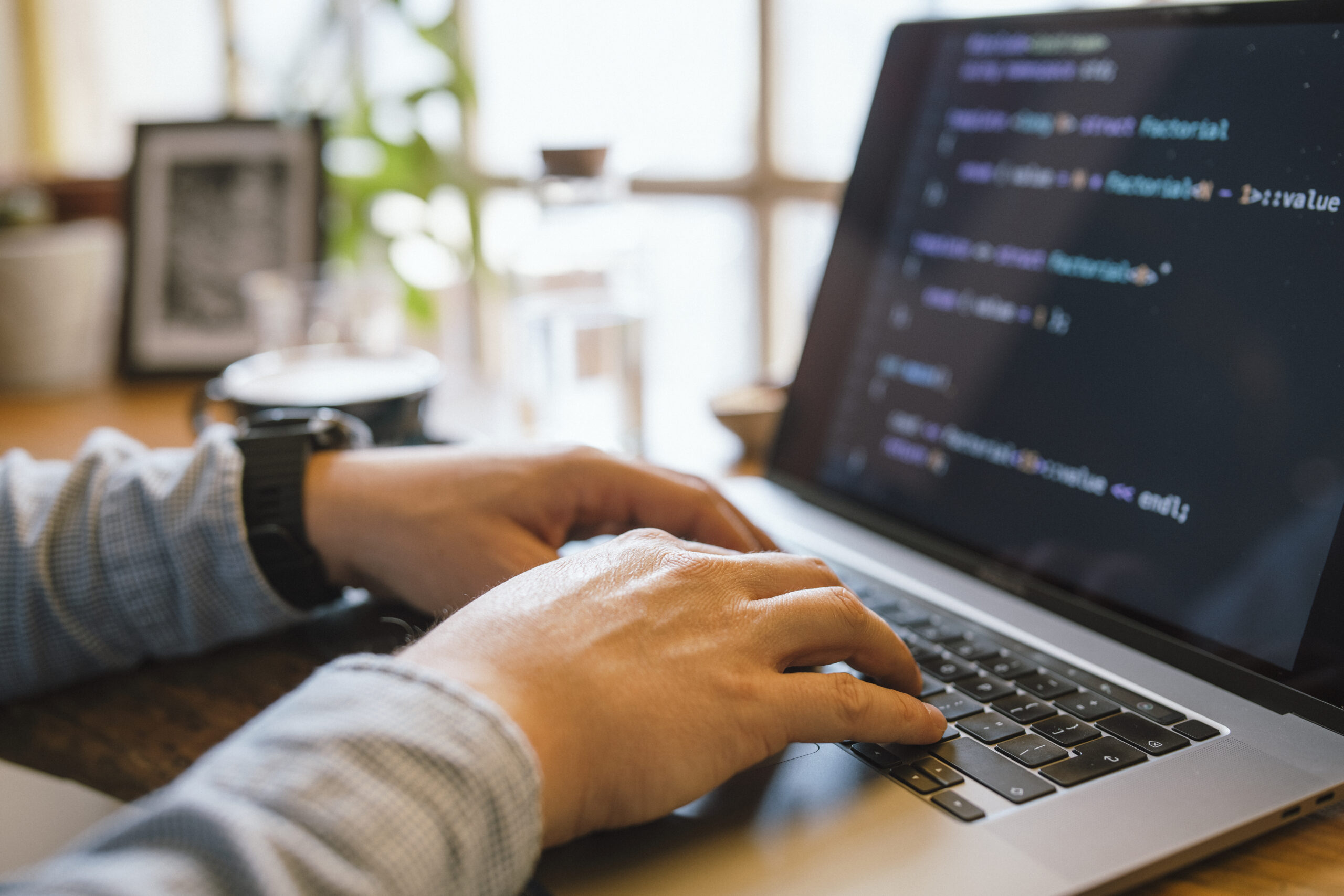
Debugging is Among the most critical — however typically forgotten — skills in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to unravel problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging techniques can help save hrs of annoyance and considerably transform your productiveness. Allow me to share quite a few procedures that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Resources
Among the quickest means builders can elevate their debugging expertise is by mastering the resources they use every single day. Although writing code is one Element of growth, realizing tips on how to communicate with it successfully during execution is Similarly crucial. Contemporary enhancement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the floor of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, step by code line by line, as well as modify code around the fly. When made use of accurately, they Permit you to observe accurately how your code behaves for the duration of execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They permit you to inspect the DOM, watch network requests, look at real-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can change disheartening UI problems into workable responsibilities.
For backend or method-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning procedures and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Variation control techniques like Git to grasp code record, locate the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your instruments usually means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your progress ecosystem so that when problems arise, you’re not misplaced at midnight. The better you already know your instruments, the greater time it is possible to commit fixing the actual difficulty in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — methods in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers will need to make a constant environment or state of affairs wherever the bug reliably appears. Without reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code adjustments.
Step one in reproducing a problem is accumulating as much context as feasible. Check with queries like: What steps triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
Once you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood environment. This might mean inputting a similar info, simulating identical consumer interactions, or mimicking process states. If the issue appears intermittently, take into consideration crafting automated assessments that replicate the edge situations or point out transitions involved. These assessments not just assistance expose the trouble but will also stop regressions Sooner or later.
Sometimes, The problem may be surroundings-unique — it might take place only on sure operating programs, browsers, or underneath certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating this kind of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for tolerance, observation, and a methodical strategy. But as soon as you can regularly recreate the bug, you happen to be now halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications extra properly, take a look at prospective fixes securely, and talk a lot more Obviously with your team or customers. It turns an abstract criticism right into a concrete obstacle — Which’s wherever developers prosper.
Go through and Recognize the Error Messages
Mistake messages will often be the most valuable clues a developer has when a thing goes Erroneous. In lieu of observing them as annoying interruptions, developers ought to discover to treat error messages as direct communications from the procedure. They normally show you just what exactly took place, wherever it transpired, and from time to time even why it took place — if you know how to interpret them.
Start by examining the concept very carefully and in complete. Lots of builders, particularly when under time tension, look at the very first line and immediately start building assumptions. But deeper within the mistake stack or logs may possibly lie the correct root cause. Don’t just duplicate and paste mistake messages into engines like google — study and have an understanding of them 1st.
Break the mistake down into elements. Can it be a syntax mistake, a runtime exception, or even a logic mistake? Will it stage to a selected file and line amount? What module or function activated it? These inquiries can guideline your investigation and level you towards the accountable code.
It’s also practical to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Discovering to recognize these can considerably speed up your debugging approach.
Some problems are vague or generic, and in All those cases, it’s vital to look at the context in which the error happened. Check connected log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings either. These usually precede larger troubles and supply hints about likely bugs.
Finally, error messages usually are not your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, serving to you pinpoint problems more rapidly, lower debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is The most powerful resources in a very developer’s debugging toolkit. When made use of effectively, it provides genuine-time insights into how an application behaves, aiding you recognize what’s going on underneath the hood without having to pause execution or action from the code line by line.
A fantastic logging tactic starts off with figuring out what to log and at what level. Common logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details through progress, Details for standard functions (like profitable start off-ups), WARN for potential challenges that don’t break the applying, Mistake for true difficulties, and FATAL when the system can’t continue.
Stay clear of flooding your logs with extreme or irrelevant facts. An excessive amount logging can obscure vital messages and decelerate your procedure. Give attention to crucial occasions, state alterations, input/output values, and demanding choice details as part of your code.
Structure your log messages Plainly and constantly. Consist of context, for example timestamps, request IDs, and performance names, so it’s simpler to trace problems in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
Through debugging, logs Permit you to monitor how variables evolve, what circumstances are achieved, and what branches of logic are executed—all without having halting This system. They’re Specially valuable in generation environments exactly where stepping via code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a well-assumed-out logging strategy, you could reduce the time it requires to identify problems, achieve further visibility into your applications, and Enhance the overall maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex task—it's a type of investigation. To correctly determine and correct bugs, builders must strategy the method similar to a detective resolving a secret. This mindset can help stop working complex problems into manageable elements and comply with clues logically to uncover the foundation induce.
Start by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much appropriate data as it is possible to devoid of leaping to conclusions. Use logs, examination situations, and consumer reviews to piece with each other a clear picture of what’s taking place.
Up coming, type hypotheses. Inquire your self: What might be causing this actions? Have any variations a short while ago been get more info designed to the codebase? Has this difficulty happened ahead of beneath equivalent circumstances? The goal is to slender down options and identify potential culprits.
Then, test your theories systematically. Try to recreate the condition in a very managed surroundings. In the event you suspect a selected purpose or element, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code concerns and Enable the final results lead you nearer to the truth.
Pay shut awareness to modest particulars. Bugs often cover within the the very least anticipated places—just like a missing semicolon, an off-by-one error, or a race affliction. Be thorough and affected individual, resisting the urge to patch the issue without the need of completely understanding it. Momentary fixes might disguise the true trouble, only for it to resurface later on.
Lastly, hold notes on Everything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for potential challenges and aid Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical capabilities, solution difficulties methodically, and develop into more effective at uncovering concealed difficulties in complicated techniques.
Produce Checks
Creating exams is among the simplest tips on how to transform your debugging competencies and General advancement effectiveness. Assessments don't just help catch bugs early but additionally serve as a safety Internet that gives you self-assurance when generating variations towards your codebase. A well-tested application is easier to debug because it permits you to pinpoint specifically the place and when a dilemma takes place.
Start with device checks, which focus on individual capabilities or modules. These small, isolated checks can immediately expose whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glimpse, appreciably cutting down enough time put in debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear just after Earlier currently being set.
Next, combine integration assessments and stop-to-finish tests into your workflow. These help make sure a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex systems with many elements or services interacting. If a little something breaks, your exams can tell you which Component of the pipeline failed and under what problems.
Creating assessments also forces you to think critically regarding your code. To test a element effectively, you need to grasp its inputs, expected outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to higher code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails regularly, it is possible to focus on repairing the bug and enjoy your examination go when the issue is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In short, composing assessments turns debugging from the irritating guessing match right into a structured and predictable method—serving to you capture more bugs, more quickly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, hoping Alternative following Answer. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and often see the issue from a new viewpoint.
When you're also near to the code for also prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this condition, your brain gets to be much less effective at issue-solving. A brief wander, a coffee break, or perhaps switching to a different task for ten–15 minutes can refresh your concentration. A lot of developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through for a longer period debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver about, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of solving it.
Study From Every Bug
Just about every bug you come upon is more than just A brief setback—It is really an opportunity to expand being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you some thing useful for those who make an effort to reflect and examine what went Erroneous.
Get started by inquiring by yourself a handful of critical questions once the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with better practices like device tests, code assessments, or logging? The responses often reveal blind spots within your workflow or knowing and allow you to Create more robust coding behaviors going ahead.
Documenting bugs will also be an excellent habit. Keep a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you discovered. As time passes, you’ll start to see styles—recurring difficulties or common mistakes—that you can proactively avoid.
In workforce environments, sharing Anything you've realized from a bug together with your friends might be Specially effective. No matter whether it’s by way of a Slack message, a brief publish-up, or a quick understanding-sharing session, helping Some others stay away from the exact same problem boosts group performance and cultivates a more robust Understanding culture.
More importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll start off appreciating them as essential portions of your advancement journey. In fact, many of the very best builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Every bug you deal with adds a whole new layer to your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.